EL表达式
概念
Expression Language 表达式语言
作用
替换和简化jsp页面中java代码的编写
语法:
注意:
jsp默认支持el表达式的。如果要忽略el表达式
- 设置jsp中
page
指令中:isELIgnored="true"
忽略当前jsp页面中所有的el表达式
\${表达式}
:忽略当前
这个el表达式
使用:
运算
运算符:
算数运算符: + - * /(div) %(mod)
比较运算符: > < >= <= == !=
逻辑运算符: &&(and) ||(or) !(not)
空运算符:empty
- 功能:用于判断字符串、集合、数组对象是否为
null
或者长度
是否为0
${empty list}:
判断字符串、集合、数组对象是否为null
或者长度
为0
${not empty str}
:表示判断字符串、集合、数组对象是否不为null
并且 长度>0
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 16:19 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <h3>算术运算符</h3> ${3+4}<br> ${3/4}<br> ${3 div 4}<br> ${3 % 4}<br> ${3 mod 4}<br> <h3>比较运算符</h3> ${3==4}<br> <h3>逻辑运算符</h3> ${3>4 && 3<4}<br> ${3>4 and 3<4}<br> </body> </html>
|
效果展示:
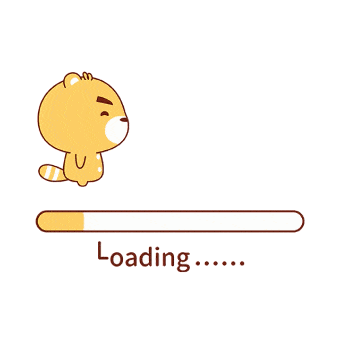
获取值
el表达式只能从域对象中获取值
语法
${域名称.键名}
从指定域中获取指定键的值
域名称:
pageScope –> pageContext
requestScope –> request
sessionScope –> session
applicationScope –> application(ServletContext)
示例:在request
域中存储了name=张三
,使用${requestScope.name}
进行获取
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 16:19 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <% request.setAttribute("name","张三"); session.setAttribute("age","23"); %>
<h3>获取值</h3> ${requestScope.name} ${sessionScope.age} </body> </html>
|
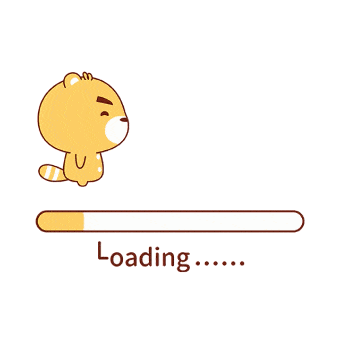
${键名}
表示依次从最小的域中查找是否有该键对应的值,直到找到为止。
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 16:19 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <html> <head> <title>Title</title> </head> <body> <% request.setAttribute("name","张三"); session.setAttribute("name","23"); %> <h3>获取值</h3> ${name} </body> </html>
|
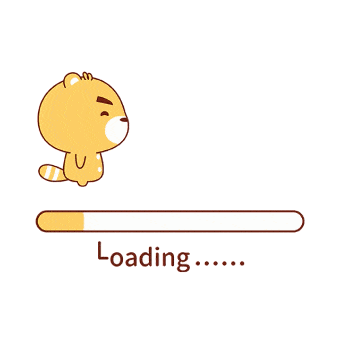
获取对象、List集合、Map集合的值
1.对象:${域名称.键名.属性名}
本质上会去调用对象的getter
方法
示例:
创建JavaBean对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| package com.uestc.domain; import java.text.SimpleDateFormat; import java.util.Date; public class User { private String name; private int age; private Date birthday;
public String getName() { return name; }
public String getBitStr(){ if(birthday!=null){ SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); return sdf.format(birthday); }else{ return ""; } } public void setName(String name) { this.name = name; } public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } }
|
创建el.jsp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 17:08 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ page import="com.uestc.domain.User" %> <%@ page import="java.util.Date" %> <html> <head> <title>Title</title> </head> <body> <% User user=new User(); user.setName("张三"); user.setAge(23); user.setBirthday(new Date()); request.setAttribute("user",user); %> <h3>使用el获取对象中的值</h3> ${requestScope.user}<br>
<%-- 通过的是对象的属性来获取 setter或getter方法,去掉set或者get,在将剩余部分首字母变为小写 setName --> Name -->name
--%> ${requestScope.user.name}<br> ${requestScope.user.age}<br> ${requestScope.user.bitStr}<br> ${requestScope.user.birthday.month}<br> </body> </html>
|
效果展示:
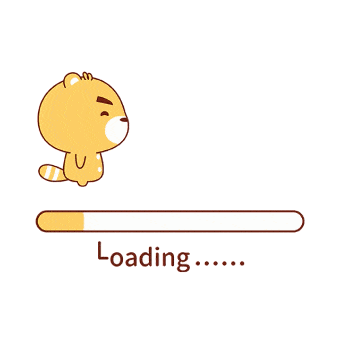
2. List集合:${域名称.键名[索引]}
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 17:08 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ page import="com.uestc.domain.User" %> <%@ page import="java.util.Date" %> <%@ page import="java.util.List" %> <%@ page import="java.util.ArrayList" %> <html> <head> <title>Title</title> </head> <body> <% User user=new User(); user.setName("张三"); user.setAge(11); user.setBirthday(new Date()); List list=new ArrayList(); list.add(1); list.add(2); list.add(3); list.add(user); request.setAttribute("list",list); %> <h3>el获取list的值</h3> ${list}<br> ${list[0]}<br> ${list[1]}<br> ${list[2]}<br> ${list[3]}<br> ${list[3].name}<br> ${list[3].age}<br> ${list[3].bitStr}<br> </body> </html>
|
显示效果:
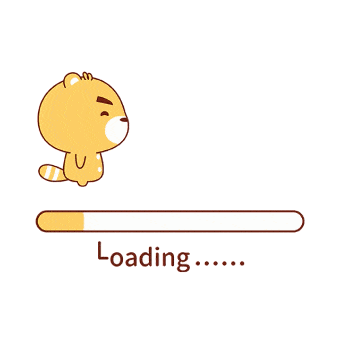
3. Map集合:
${域名称.键名.key名称}
${域名称.键名["key名称"]}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 17:08 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ page import="com.uestc.domain.User" %> <%@ page import="java.util.*" %> <html> <head> <title>Title</title> </head> <body> <% User user=new User(); user.setName("张三"); user.setAge(11); user.setBirthday(new Date()); Map map=new HashMap(); map.put("sname","李四"); map.put("gender","男"); map.put("user",user); request.setAttribute("map",map); %> <h3>el获取map的值</h3> ${map.gender}<br> ${map["gender"]}<br> ${map.sname}<br> ${map["sname"]}<br> ${map.user.name} </body> </html>
|
效果展示:
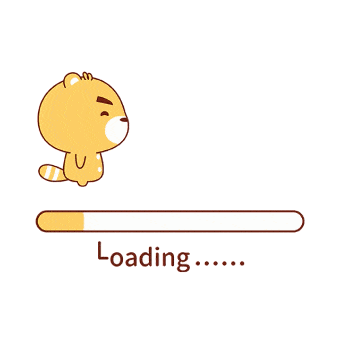
隐式对象
el表达式中有11个隐式对象
pageContext
:
获取jsp
其他八个内置对象
1
| ${pageContext.request.contextPath}:动态获取虚拟目录JSTL
|