JSTL
概念
JavaServer Pages Tag Library JSP标准标签库
是由Apache组织提供的开源的免费的jsp标签 <标签>
作用
用于简化和替换jsp页面上的java代码
使用步骤
导入jstl
相关jar
包
引入标签库:taglib
指令: <%@ taglib %>
使用标签
示例:
1.导入jstl
相关jar
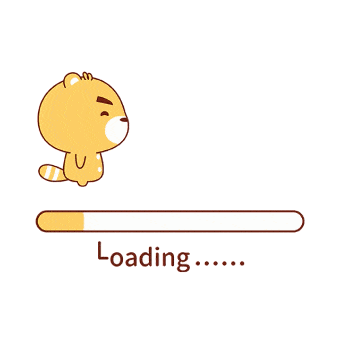
引入标签库:taglib
指令: <%@ taglib %>
1
| <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
|
常用的JSTL标签
if
:
相当于java代码的if
语句
属性:test
必须属性,接受boolean
表达式
- 如果表达式为
true
,则显示if
标签体内容,如果为false,则不显示标签体内容
- 一般情况下,
test
属性值会结合el表达式
一起使用
注意:
- c:if标签没有
else
情况,想要else情况,则可以在定义一个c:if
标签
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| <%@ page import="java.util.ArrayList" %> <%@ page import="java.util.List" %><%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 22:27 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% List list=new ArrayList(); list.add("aaa"); request.setAttribute("list",list);
request.setAttribute("number",3); %> <c:if test="${not empty list}"> 遍历集合 </c:if> <br> <c:if test="${number %2 !=0}"> ${number}为奇数 </c:if> <c:if test="${number %2==0}"> ${number}为偶数 </c:if> </body> </html>
|
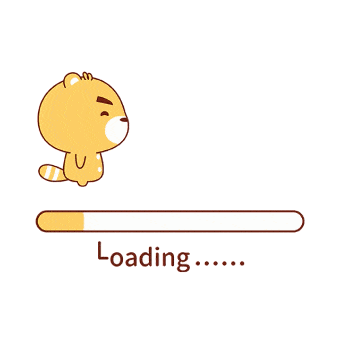
choose
:
相当于java代码的switch
语句
使用choose标签声明 相当于switch声明
使用when标签做判断 相当于case
使用otherwise标签做其他情况的声明 相当于default
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 23:38 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>choose标签</title> </head> <body> <%--完成数字编号对应星期几的案例 1. 使用choose标签声明 相当于switch声明 2. 使用when标签做判断 相当于case 3. 使用otherwise标签做其他情况的声明 相当于default --%> <% request.setAttribute("number",3); %> <c:choose> <c:when test="${number==1}">星期一</c:when> <c:when test="${number==2}">星期二</c:when> <c:when test="${number==3}">星期三</c:when> <c:when test="${number==4}">星期四</c:when> <c:when test="${number==5}">星期五</c:when> <c:when test="${number==6}">星期六</c:when> <c:when test="${number==7}">星期日</c:when> <c:otherwise>数字有误</c:otherwise> </c:choose> </body> </html>
|
foreach
相当于java代码的for
语句
完成重复的操作
属性:
begin
:开始值
end
:结束值
var
:临时变量
step
:步长
varStatus
:循环状态对象
index
:容器中元素的索引,从0
开始
count
:循环次数,从1
开始
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 23:52 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <c:forEach begin="1" end="10" var="i" step="2" varStatus="s"> ${i} ${s.index} ${s.count}<br> </c:forEach> </body> </html>
|
效果展示:
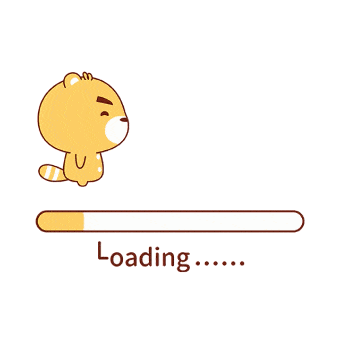
遍历容器
1 2
| List<User> list; for(User u:list){}
|
示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <%@ page import="java.util.ArrayList" %> <%@ page import="java.util.List" %><%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/3 Time: 23:52 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% List list=new ArrayList(); list.add("aaa"); list.add("bbb"); list.add("ccc"); request.setAttribute("list",list); %> <c:forEach items="${list}" var="i" varStatus="s"> ${i} ${s.index} ${s.count}<br> </c:forEach> </body> </html>
|
效果展示:
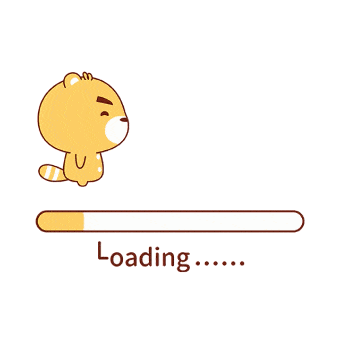
小练习
需求:
在request域中有一个存有User对象的List集合。需要使用jstl+el将list集合数据展示到jsp页面的表格table中
- 新建
User
类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| package com.uestc.domain;
import java.text.SimpleDateFormat; import java.util.Date;
public class User { private String name; private int age; private Date birthday;
public User(String name, int age, Date birthday) { this.name = name; this.age = age; this.birthday = birthday; } public User(){
}
public String getBitStr(){ if(birthday!=null){ SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); return sdf.format(birthday); }else{ return ""; } } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public Date getBirthday() { return birthday; } public void setBirthday(Date birthday) { this.birthday = birthday; } }
|
- 新建
jstl_test.jsp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| <%@ page import="java.util.ArrayList" %> <%@ page import="java.util.List" %><%-- Created by IntelliJ IDEA. User: Liu Fei Date: 2020/11/4 Time: 0:21 To change this template use File | Settings | File Templates. --%> <%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ page import="com.uestc.domain.User" %> <%@ page import="java.util.Date" %> <%@ taglib prefix="" uri="http://java.sun.com/jsp/jstl/core" %> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Title</title> </head> <body> <% List list=new ArrayList(); list.add(new User("张三",23,new Date())); list.add(new User("李四",24,new Date())); list.add(new User("王五",26,new Date())); request.setAttribute("list",list); %> <table border="1" width="500" align="left"> <tr> <th>编号</th> <th>姓名</th> <th>年龄</th> <th>生日</th> </tr> <%--数据行 偶数行呈现 --%> <c:forEach items="${list}" var="user" varStatus="s"> <c:if test="${s.count %2==0}"> <tr bgcolor="#a9aW9a9"> <td>${s.count}</td> <td>${user.name}</td> <td>${user.age}</td> <td>${user.bitStr}</td> </tr> </c:if> <c:if test="${s.count %2!=0}"> <tr bgcolor="#f0ffff"> <td>${s.count}</td> <td>${user.name}</td> <td>${user.age}</td> <td>${user.bitStr}</td> </tr> </c:if> </c:forEach> </table> </body> </html>
|
- 效果展示
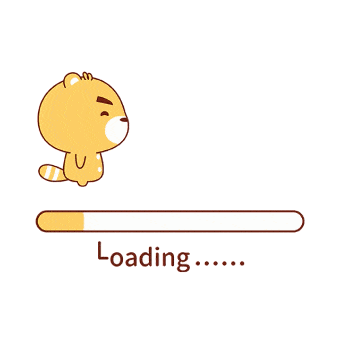