[TOC]
C下FFmpeg开发环境的搭建
1、新建VC项目
新建->项目->控制台应用->创建
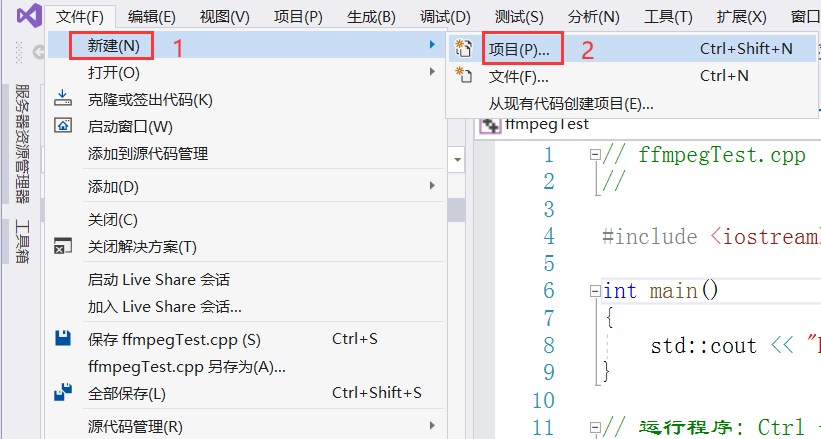
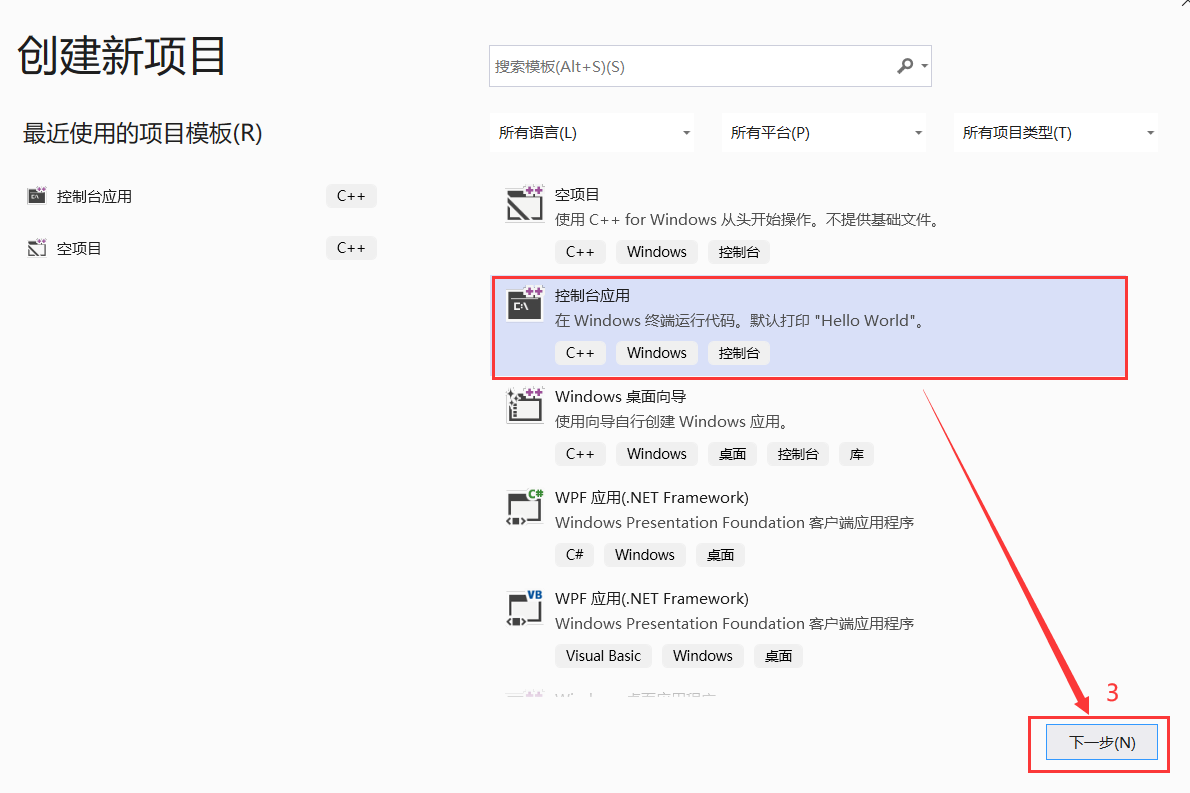
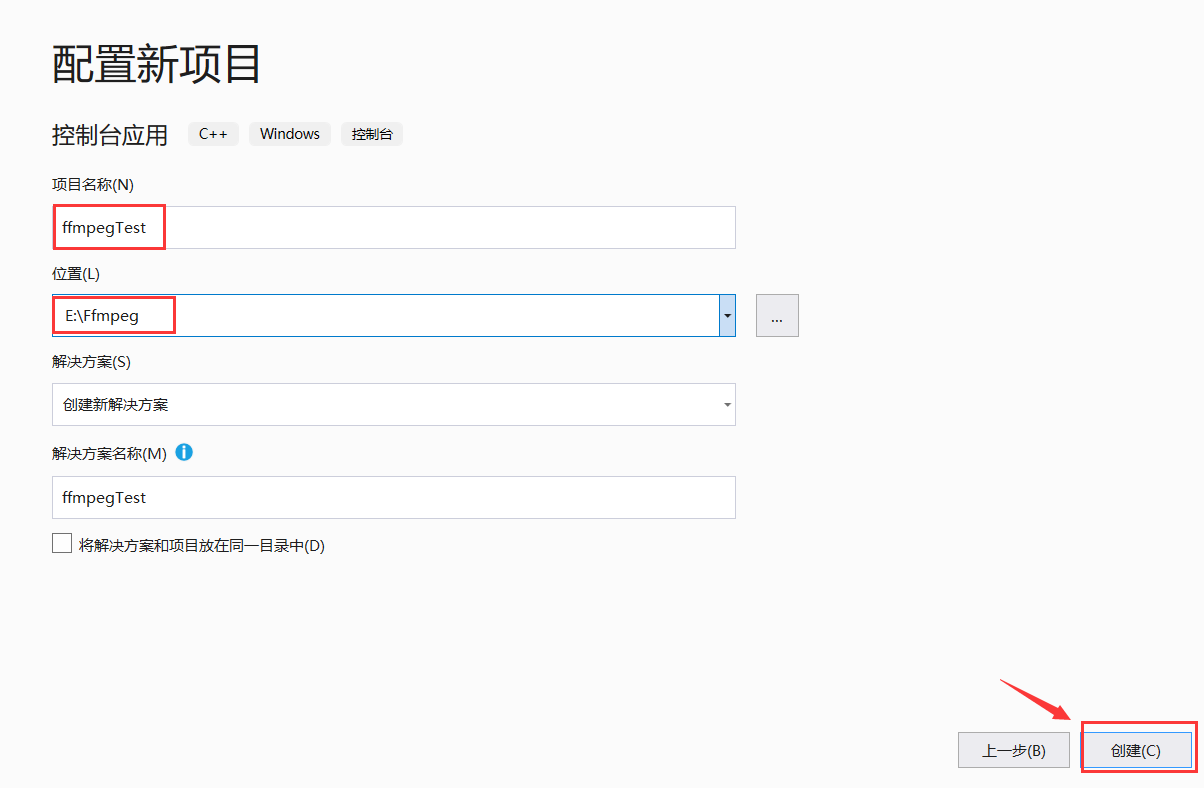
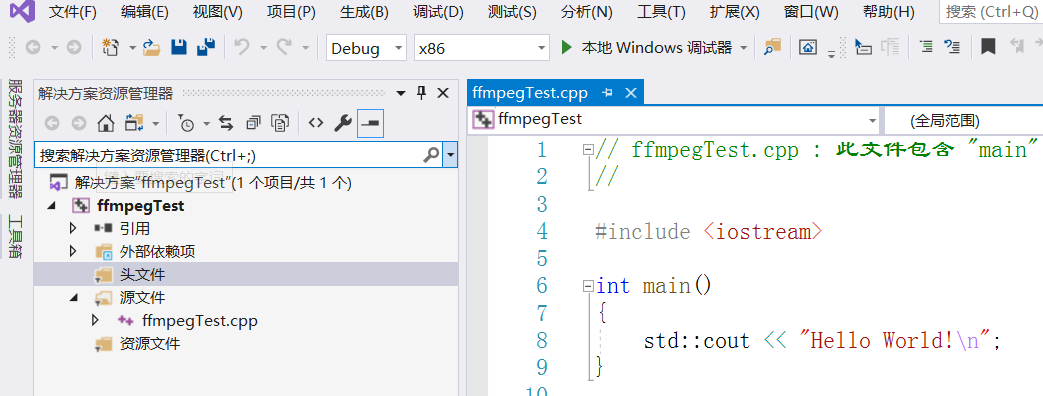
2、拷贝FFmpeg开发文件
1 | 1、头文件(*.h)拷贝至项目文件夹的include子文件夹下 |
PS:如果直接使用官网上下载的FFmpeg开发文件。则可能还需要将MinGW安装目录中的inttypes.h,stdint.h,_mingw.h三个文件拷贝至项目文件夹的include子文件夹下。
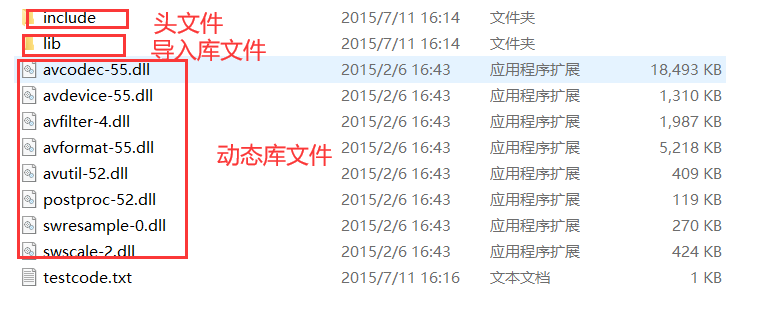
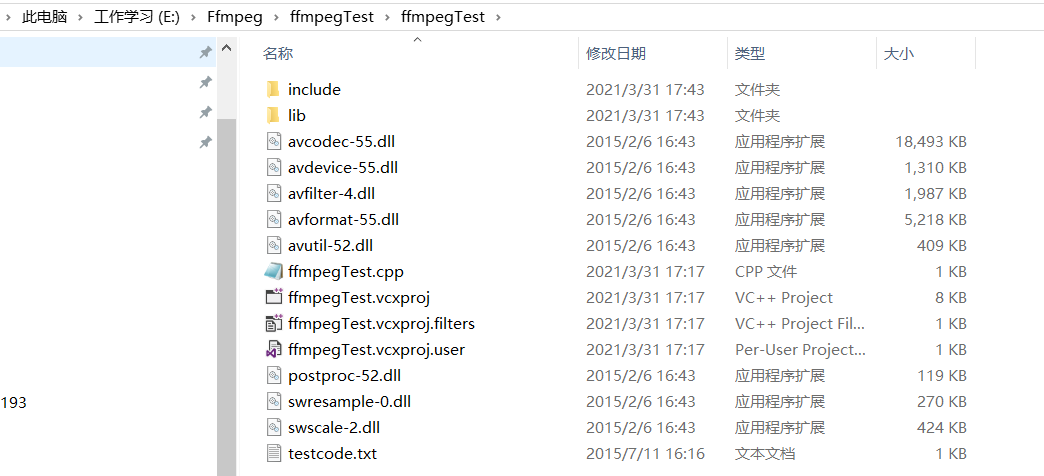
3、配置开发文件
step1: 打开属性面板
解决方案资源管理器->右键单击项目->属性
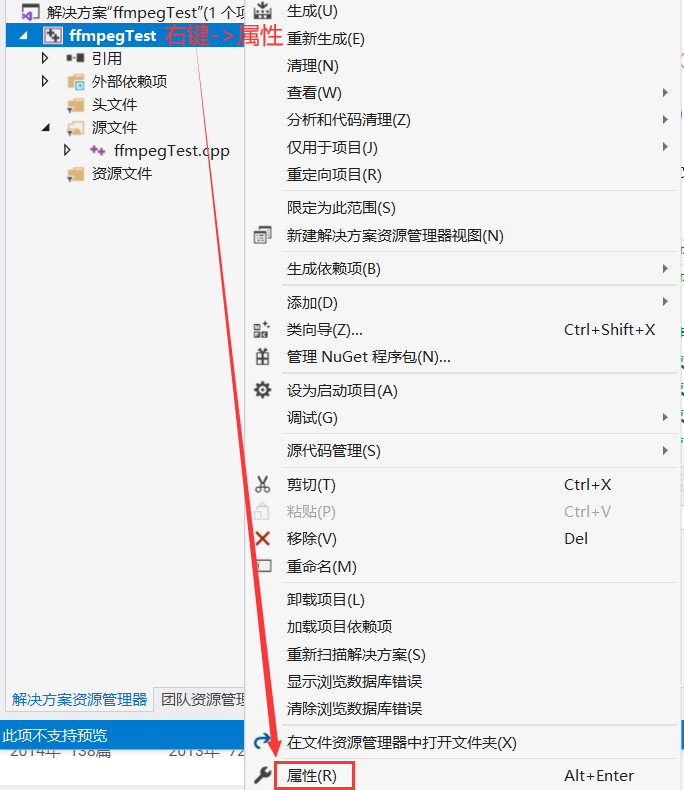
step2: 头文件配置
配置属性->C/C++->常规->附加包含目录,输入“include”(刚才拷贝头文件的目录)
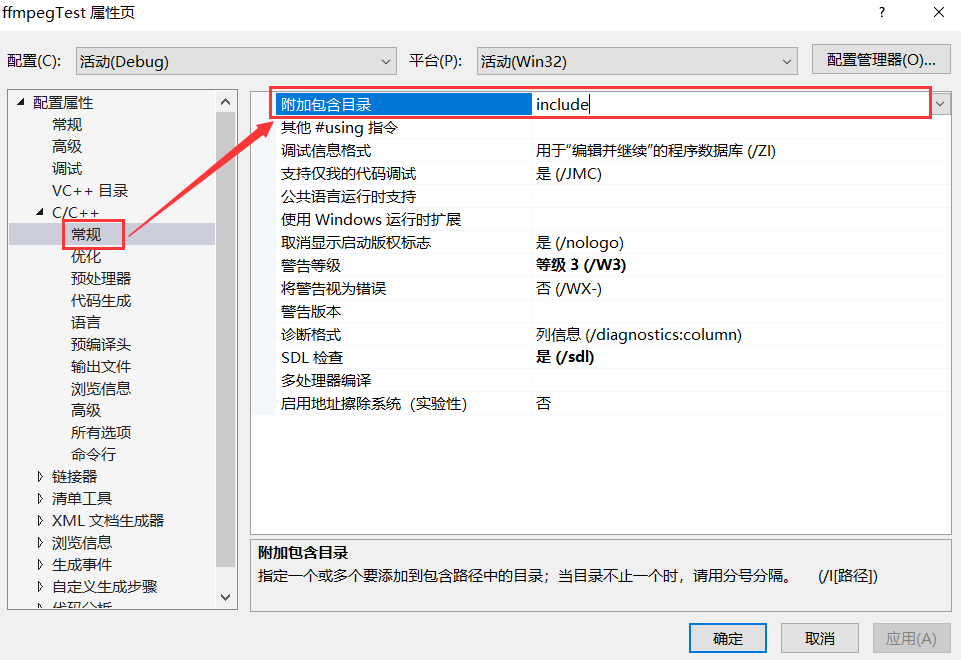
step3: 导入库配置
配置属性->链接器->常规->附加库目录,输入“lib” (刚才拷贝库文件的目录)
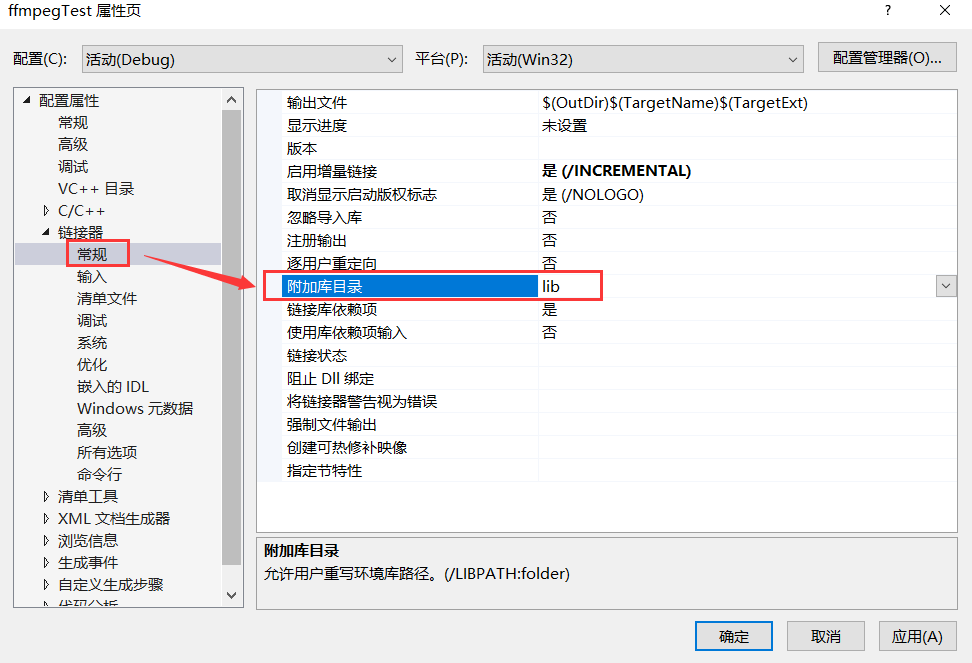
配置属性->链接器->输入->附加依赖项,输入“avcodec.lib; avformat.lib; avutil.lib; avdevice.lib; avfilter.lib; postproc.lib; swresample.lib; swscale.lib”(导入库的文件名)
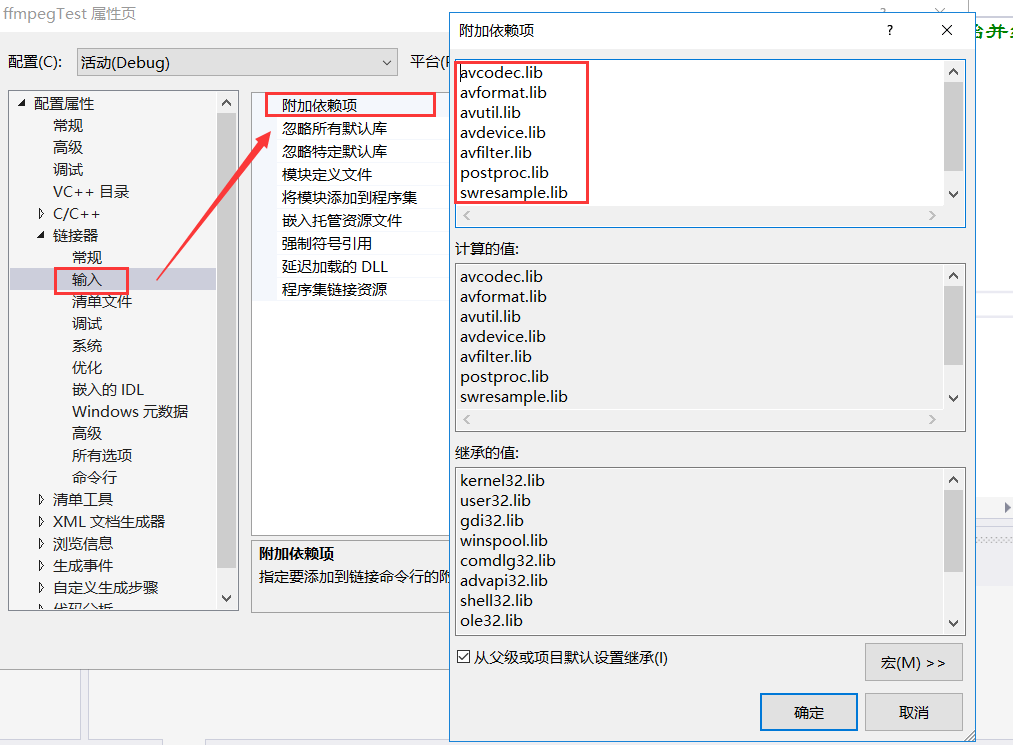
动态库不用配置
4、测试
step1: 创建源代码文件
在工程中创建一个包含main()函数的C/C++文件(如果已经有了可以跳过这一步)。
step2: 包含头文件
- 如果是C语言中使用FFmpeg,则直接使用下面代码
1 |
|
- 如果是C++语言中使用FFmpeg,则使用下面代码
1 |
|
如果运行无误,则代表FFmpeg已经配置完成。
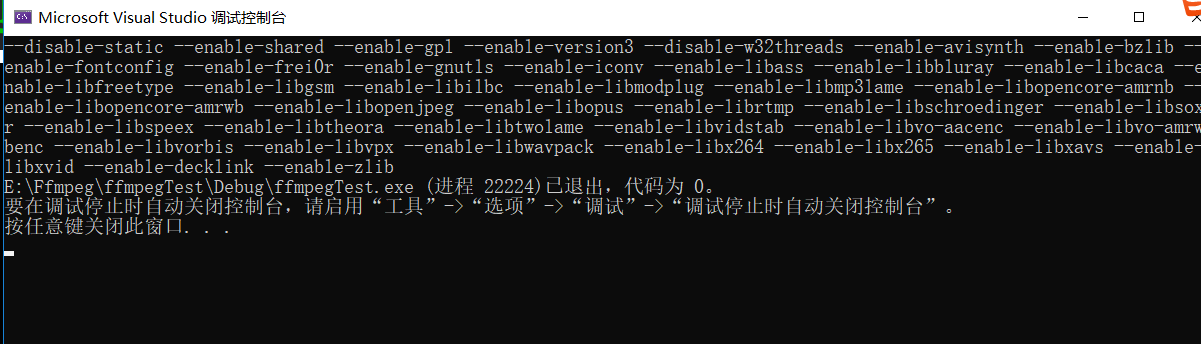
FFmpeg库简介
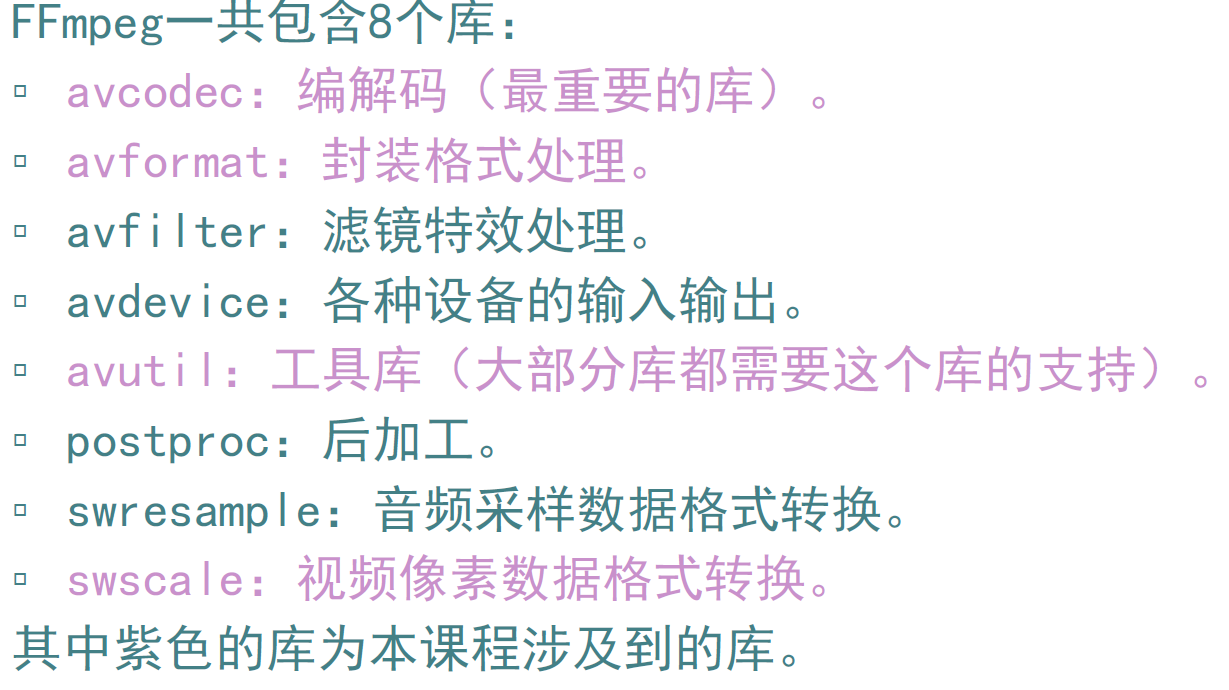
FFmpeg解码的函数
FFmpeg解码的流程图如下所示:
FFmpeg解码函数简介
1 | av_register_all():注册所有组件。 |
FFmpeg解码的数据结构
FFmpeg解码的数据结构如下所示:
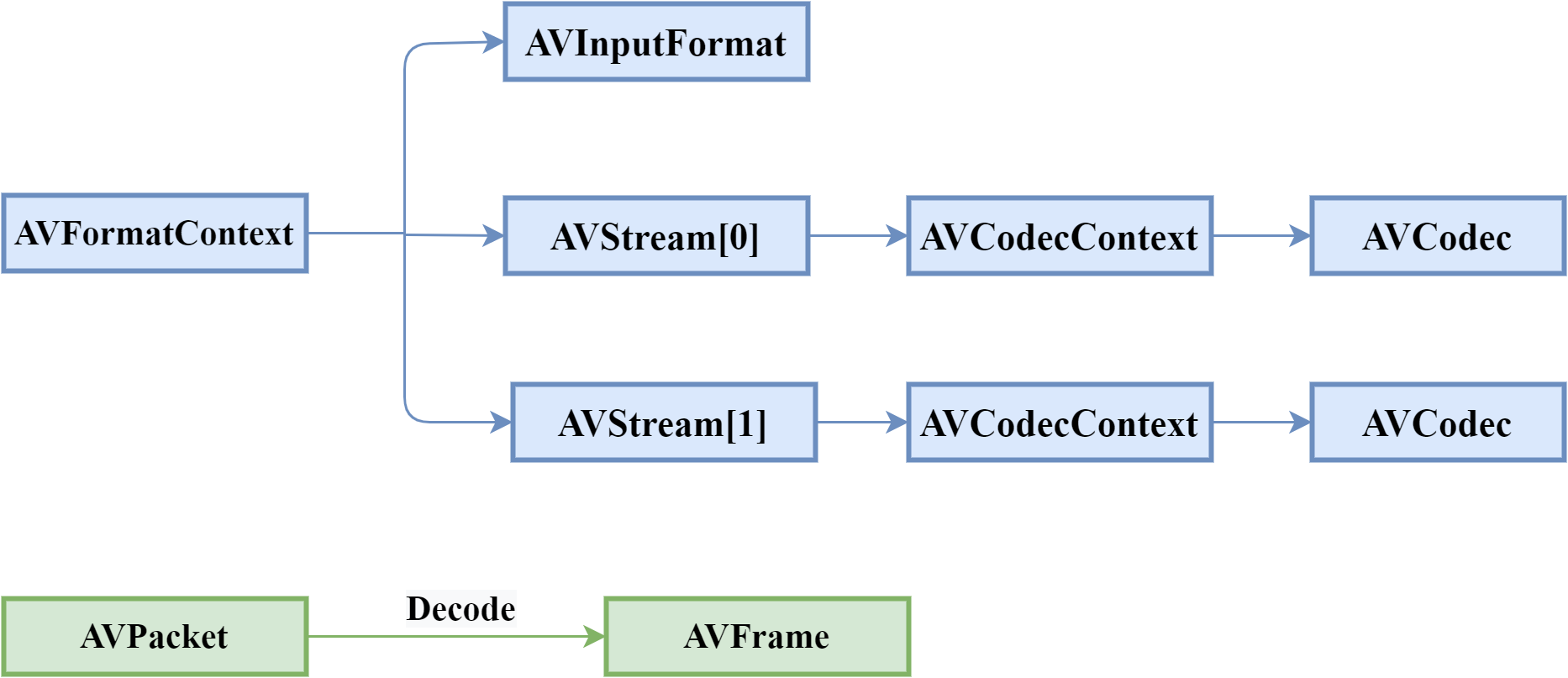
FFmpeg数据结构简介
1、AVFormatContext
封装格式上下文结构体,也是统领全局的结构体,保存了视频文件封装格式相关信息。
2、AVInputFormat
每种封装格式(例如FLV, MKV, MP4, AVI)对应一个该结构体。
3、AVStream
视频文件中每个视频(音频)流对应一个该结构体。
4、AVCodecContext
编码器上下文结构体,保存了视频(音频)编解码相关信息。
5、AVCodec
每种视频(音频)编解码器(例如H.264解码器)对应一个该结构体。
6、AVPacket
存储一帧压缩编码数据。
7、AVFrame
存储一帧解码后像素(采样)数据。
FFmpeg数据结构分析
AVFormatContext:
iformat:输入视频的AVInputFormat
nb_streams :输入视频的AVStream 个数
streams :输入视频的AVStream []数组
duration :输入视频的时长(以微秒为单位)
bit_rate:输入视频的码率
AVInputFormat:
name:封装格式名称
long_name:封装格式的长名称
extensions:封装格式的扩展名
id:封装格式ID
一些封装格式处理的接口函数AVStream:
id:序号
codec:该流对应的AVCodecContext
time_base:该流的时基
r_frame_rate:该流的帧率AVCodecContext:
codec:编解码器的AVCodec
width, height:图像的宽高(只针对视频)
pix_fmt:像素格式(只针对视频)
sample_rate:采样率(只针对音频)
channels:声道数(只针对音频)
sample_fmt:采样格式(只针对音频)AVCodec:
codec:编解码器的AVCodec
width, height:图像的宽高(只针对视频)
pix_fmt:像素格式(只针对视频)
sample_rate:采样率(只针对音频)
channels:声道数(只针对音频)
sample_fmt:采样格式(只针对音频)
AVPacket:
pts:显示时间戳
dts :解码时间戳
data :压缩编码数据
size :压缩编码数据大小
stream_index :所属的AVStream
AVFrame:
data:解码后的图像像素数据(音频采样数据)。
linesize:对视频来说是图像中一行像素的大小;对音频来说是整个音频帧的大小。
width, height:图像的宽高(只针对视频)。
key_frame:是否为关键帧(只针对视频) 。
pict_type:帧类型(只针对视频) 。例如I,P,B。
最简单的基于FFmpeg的解码器
测试文件
1 | /** |
练习
需求:
修改源代码。对于测试文件,输出以下几种文件
1、“output.h264”文件
解码前的H.264码流数据(只对MPEG-TS,AVI格式作要求)
2、“output.yuv”文件
解码后的YUV420P像素数据
3、“output.txt”文件
- 封装格式参数:封装格式、比特率、时长
- 视频编码参数:编码方式、宽高
- 每一个解码前视频帧参数:帧大小
- 每一个解码后视频帧参数:帧类型
完整代码:
1 | /** |
效果演示:
1、通过数据查看工具:YUV Player查看输出的YUV的Y数据,即只有亮度,没有色度信息,因此视频成黑白色。
2、通过数据查看工具:YUV Player查看输出的YUV数据,包括亮度和色度。
3、输出“output.txt”文件